ComponentGeneric Class Referenceabstract
#include <ComponentGeneric.h>
Inheritance diagram for ComponentGeneric:
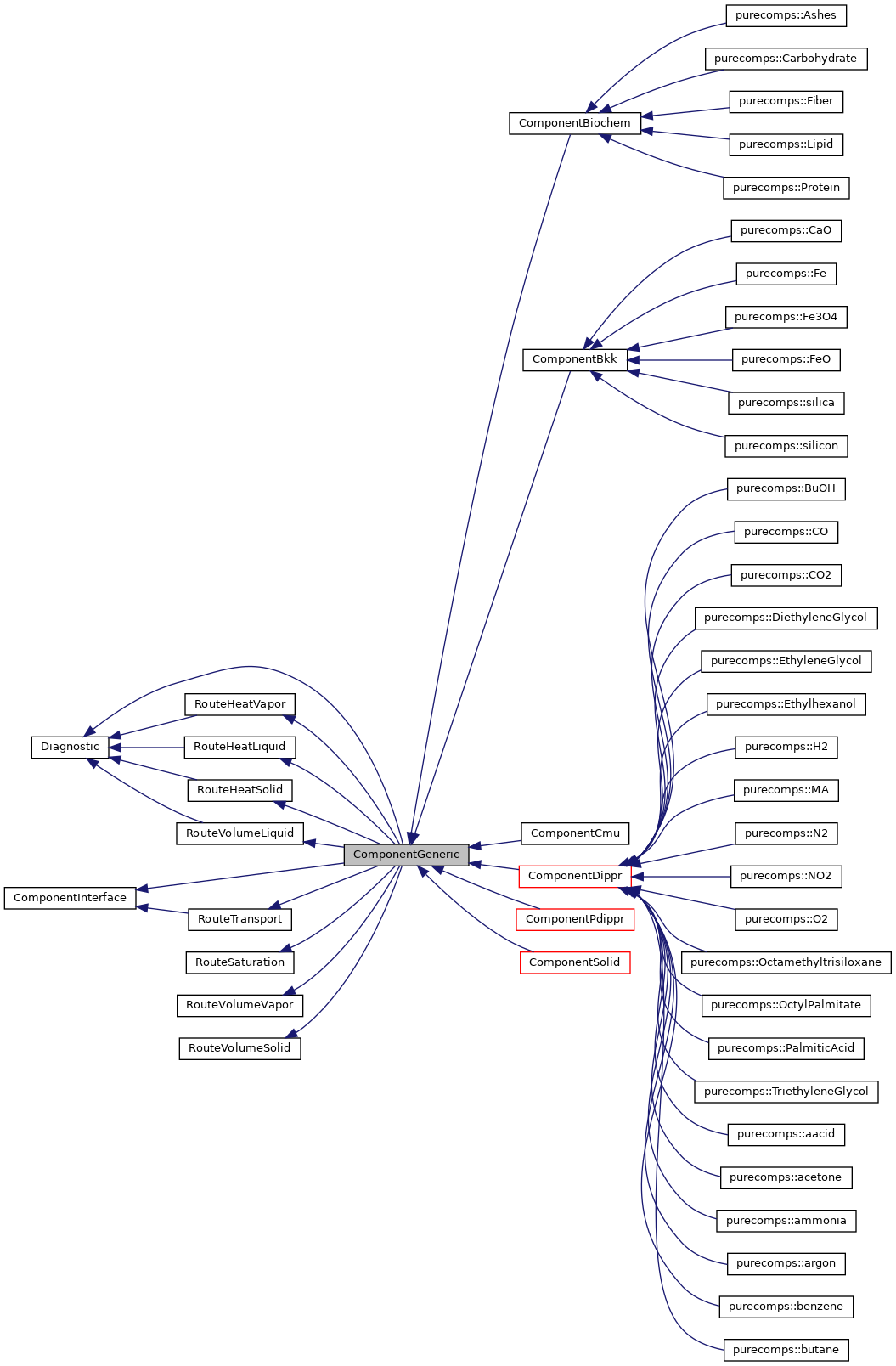
Public Member Functions | |
ComponentGeneric (std::string name) | |
virtual | ~ComponentGeneric (void) |
const std::string & | name (void) const |
int | id (void) const |
void | setid (int idd) |
virtual Value | kvli (const Value &T, const Value &P) |
virtual bool | isSolid (void)=0 |
virtual bool | isGas (void) |
![]() | |
Diagnostic (void) | |
void | setVerbosity (int verbosity) |
sets the verbosity level for this instance More... | |
int | verbosity (void) const |
![]() | |
virtual const Value & | MW (void) const =0 |
virtual const Value & | Pc (void) const =0 |
virtual const Value & | Tc (void) const =0 |
virtual const Value | Vc (void) const =0 |
virtual const Value & | Zc (void) const =0 |
virtual const Value & | omega (void) const =0 |
virtual const Value & | diffvol (void) const =0 |
virtual const Value & | vb (void) const =0 |
virtual const Value & | dh0 (void) const =0 |
virtual const Value & | dg0 (void) const =0 |
![]() | |
RouteSaturation (void) | |
virtual | ~RouteSaturation (void) |
virtual Value | psat (const Value &T, double *derT=nullptr, double *der2T=nullptr)=0 |
Vapour pressure of saturation. More... | |
virtual Value | dhvl (const Value &T, double *derT=nullptr)=0 |
Vapour-Liquid enthalpy difference. More... | |
![]() | |
RouteHeatVapor (void) | |
virtual | ~RouteHeatVapor (void) |
virtual Value | hv (const Value &T, const Value &P)=0 |
Molar vapor enthalpy, J/kmol. More... | |
virtual Value | sv (const Value &T, const Value &P)=0 |
Molar vapor entropy, J/kmol/K. More... | |
Value | gv (const Value &T, const Value &P) |
Molar vapor Gibbs free energy, J/kmol. More... | |
virtual Value | cpv (const Value &T, const Value &P)=0 |
Molar vapor cp, J/kmol/K. More... | |
![]() | |
RouteHeatLiquid (void) | |
virtual | ~RouteHeatLiquid (void) |
virtual Value | hl (const Value &T, const Value &P)=0 |
Molar liquid enthalpy, J/kmol. More... | |
virtual Value | sl (const Value &T, const Value &P)=0 |
Molar liquid entropy, J/kmol/K. More... | |
Value | gl (const Value &T, const Value &P) |
Molar liquid Gibbs free energy, J/kmol. More... | |
virtual Value | cpl (const Value &T, const Value &P)=0 |
Molar liquid cp, J/kmol/K. More... | |
![]() | |
RouteHeatSolid (void) | |
virtual | ~RouteHeatSolid (void) |
virtual Value | hs (const Value &T, const Value &P)=0 |
Molar solid enthalpy, J/kmol. More... | |
virtual Value | ss (const Value &T, const Value &P)=0 |
Molar solid entropy, J/kmol/K. More... | |
Value | gs (const Value &T, const Value &P) |
Molar solid Gibbs free energy, J/kmol. More... | |
virtual Value | cps (const Value &T, const Value &P)=0 |
Molar solid cp, J/kmol/K. More... | |
![]() | |
RouteTransport (void) | |
virtual | ~RouteTransport (void) |
virtual Value | muv (const Value &T, const Value &P)=0 |
Vapour viscosity. More... | |
virtual Value | mul (const Value &T)=0 |
Liquid viscosity. More... | |
virtual Value | kv (const Value &T, const Value &P)=0 |
Vapour thermal conductivity. More... | |
virtual Value | kl (const Value &T)=0 |
Liquid thermal conductivity. More... | |
virtual Value | sigma (const Value &T)=0 |
Liquid surface tension. More... | |
Value | DG (const Value &T, const Value &P, const Value &mixMW, const Value &mixDiffvol) |
Gas diffusivity. More... | |
Value | DL (const Value &T) |
Liquid diffusivity. More... | |
![]() | |
RouteVolumeVapor (void) | |
virtual | ~RouteVolumeVapor (void) |
virtual double | p (const double rho, const double T, double *derRho=nullptr, double *derT=nullptr)=0 |
Vapor pressure, Pa. Requires the actual implementation of the volumetric properties. More... | |
Value | p (const Value &rrho, const Value &TT) |
Vapor pressure, Pa. More... | |
virtual Value | rhov (const Value &T, const Value &P, double *derT=nullptr, double *derP=nullptr)=0 |
Molar vapor density, kmol/m3. More... | |
![]() | |
RouteVolumeLiquid (void) | |
virtual | ~RouteVolumeLiquid (void) |
virtual Value | rhol (const Value &T, const Value &P, double *derT=nullptr)=0 |
Molar liquid density, kmol/m3. More... | |
![]() | |
virtual Value | rhos (const Value &T, const Value &P, double *derT=nullptr)=0 |
Molar solid density, kmol/m3. More... | |
Additional Inherited Members | |
![]() | |
virtual | ~Diagnostic ()=default |
![]() | |
virtual | ~ComponentInterface ()=default |
![]() | |
RouteTransport (const RouteTransport &) | |
not implemented, making class non copyable More... | |
RouteTransport & | operator= (const RouteTransport &) |
not implemented, making class non copyable More... | |
![]() | |
virtual | ~RouteVolumeSolid ()=default |
![]() | |
int | verbosityInstance |
Detailed Description
User interface used by user Used in ListComponent, called by ListComponent::operator[]
#include <libpf/components/ComponentGeneric.h>
- Remarks
- Warning
- Thread safe:
- Extendable:
- Platform dependencies:
Constructor & Destructor Documentation
◆ ComponentGeneric()
|
explicit |
- Parameters
-
name default component indentifier
◆ ~ComponentGeneric()
|
inlinevirtual |
Member Function Documentation
◆ id()
int ComponentGeneric::id | ( | void | ) | const |
◆ isGas()
|
virtual |
Check function for incondensable component (i.e. with activity reference state based on the asymmetric convention)
- Returns
- true if a component is gas
- false otherwise
Reimplemented in ComponentDippr, and ComponentPdippr.
◆ isSolid()
|
pure virtual |
Check function for solid component
- Returns
- true if a component is solid
- false otherwise
Implemented in ComponentBiochem, ComponentBkk, ComponentDippr, ComponentPdippr, and ComponentSolid.
◆ kvli()
Vapor-liquid equilibrium constant
- Parameters
-
T temperature K P pressure Pa
- Returns
- Vapor-liquid equilibrium constant
Reimplemented in ComponentBiochem, ComponentBkk, ComponentDippr, ComponentPdippr, and ComponentSolid.
◆ name()
const std::string & ComponentGeneric::name | ( | void | ) | const |
Introspection function
Can be used to ask/check the name of an object.
Can be used to set the name of an object.
- Returns
- a reference to the string of the object instance name
◆ setid()
void ComponentGeneric::setid | ( | int | idd | ) |
The documentation for this class was generated from the following file: